Home Documentation Writing Reading Pictures1 Pictures2 Formulas DateTime SheetByName
Merging Grouping InsertRowCol NumFormats Formats Fonts Buffer1 Buffer2 Copying TopNFilter
Sorting StringFilter NumberFilter FilterByValues Protection Replacing RichString BeginWith
ColorScale OpRule AltRows
Merging Grouping InsertRowCol NumFormats Formats Fonts Buffer1 Buffer2 Copying TopNFilter
Sorting StringFilter NumberFilter FilterByValues Protection Replacing RichString BeginWith
ColorScale OpRule AltRows
Customizing fonts
This example shows how to use fonts. See the result in the fonts.xlsx file.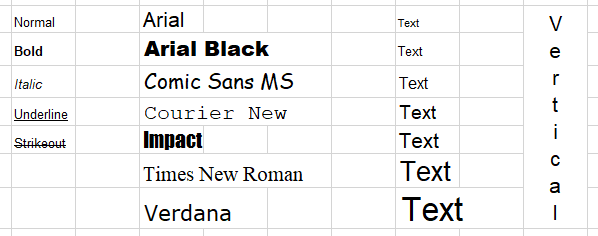
#include "libxl.h" using namespace libxl; int main() { Book* book = xlCreateXMLBook(); Sheet* sheet = book->addSheet(L"Sheet1"); const wchar_t* fonts[] = {L"Arial", L"Arial Black", L"Comic Sans MS", L"Courier New", L"Impact", L"Times New Roman", L"Verdana"}; for(int i = 0; i < sizeof(fonts) / sizeof(const wchar_t*); ++i) { Font* font = book->addFont(); font->setSize(16); font->setName(fonts[i]); Format* format = book->addFormat(); format->setFont(font); sheet->writeStr(i + 2, 3, fonts[i], format); } int fontSize[] = {8, 10, 12, 14, 16, 20, 25}; for(int i = 0; i < sizeof(fontSize) / sizeof(int); ++i) { Font* font = book->addFont(); font->setSize(fontSize[i]); Format* format = book->addFormat(); format->setFont(font); sheet->writeStr(i + 2, 7, L"Text", format); } Font* font = book->addFont(); font->setSize(16); Format* format = book->addFormat(); format->setRotation(255); format->setFont(font); sheet->writeStr(2, 9, L"Vertical", format); sheet->setMerge(2, 8, 9, 9); Font* boldFont = book->addFont(); boldFont->setBold(); Format* boldFormat = book->addFormat(); boldFormat->setFont(boldFont); Font* italicFont = book->addFont(); italicFont->setItalic(); Format* italicFormat = book->addFormat(); italicFormat->setFont(italicFont); Font* underlineFont = book->addFont(); underlineFont->setUnderline(UNDERLINE_SINGLE); Format* underlineFormat = book->addFormat(); underlineFormat->setFont(underlineFont); Font* strikeoutFont = book->addFont(); strikeoutFont->setStrikeOut(); Format* strikeoutFormat = book->addFormat(); strikeoutFormat->setFont(strikeoutFont); sheet->writeStr(2, 1, L"Normal"); sheet->writeStr(3, 1, L"Bold", boldFormat); sheet->writeStr(4, 1, L"Italic", italicFormat); sheet->writeStr(5, 1, L"Underline", underlineFormat); sheet->writeStr(6, 1, L"Strikeout", strikeoutFormat); book->save(L"fonts.xlsx"); book->release(); return 0; }