Home Documentation Writing Reading Pictures1 Pictures2 Formulas Date/Time SheetByName
Merging Grouping InsertRowCol NumFormats Formats Fonts Buffer1 Buffer2 Copying TopNFilter
Sorting StringFilter NumberFilter FilterByValues Protection Replacing RichString BeginWith
ColorScale OpRule AltRows
Merging Grouping InsertRowCol NumFormats Formats Fonts Buffer1 Buffer2 Copying TopNFilter
Sorting StringFilter NumberFilter FilterByValues Protection Replacing RichString BeginWith
ColorScale OpRule AltRows
Conditional formatting: highlighting cells that begin with the given text
This example defines a conditional format with a bold font and sets conditional formatting for the range B3:B11 for highlighting cells that begin with 'a'.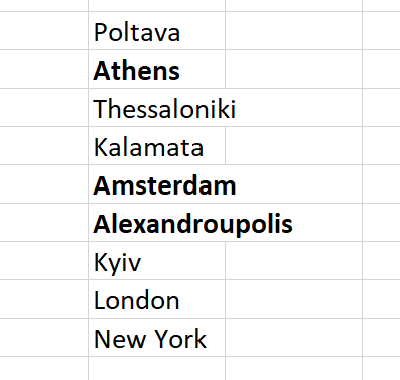
#include "libxl.h" using namespace libxl; int main() { Book* book = xlCreateXMLBook(); Sheet* sheet = book->addSheet(L"my"); sheet->writeStr(2, 1, L"Poltava"); sheet->writeStr(3, 1, L"Athens"); sheet->writeStr(4, 1, L"Thessaloniki"); sheet->writeStr(5, 1, L"Kalamata"); sheet->writeStr(6, 1, L"Amsterdam"); sheet->writeStr(7, 1, L"Alexandroupolis"); sheet->writeStr(8, 1, L"Kyiv"); sheet->writeStr(9, 1, L"London"); sheet->writeStr(10, 1, L"New York"); ConditionalFormat* cFormat = book->addConditionalFormat(); cFormat->font()->setBold(true); ConditionalFormatting* cf = sheet->addConditionalFormatting(); cf->addRange(2, 10, 1, 1); cf->addRule(CFORMAT_BEGINWITH, cFormat, L"a"); book->save(L"out.xlsx"); book->release(); return 0; }
See also: Highlighting cells that begin with the given text
Creating a gradated color scale on the cells
Highlighting cells that more than the specified value
Highlighting alternating rows